In this tutorial, I’m going to start the process of making a prototype using mock data. My goal at the end of the series is to have quickly mocked data for a prototype while binding it to the UI. As a result, the data can be used across the app and there is only one point of maintenance.
When I’m done with the series, I will have one data set that I can use to create demo components of my application.
Quick note…there are libraries out there that do this for you. I believe in my bones that learning to do it first manually will really help you understand the data you are designing for. Therefore, It will also give you a better foundation to solve any problems that pop up. I always advocate doing a little bit before involving a library to automate the process.

How do I use this at work?
If you are a front-end developer or designer who codes and working without the back-end API for a proof of concept or prototype in the browser, this is for you. In order to complete this tutorial, you’ll need to have a good understanding of HTML. And how to start an Angular application.
The skills in this series allow you to create demo content in your prototype. This is really useful for other teams within your organization that need to see real world data. You can also use it to make final design adjustments based on real data.
Often in my role as a designer & developer I am working way early in the process. I don’t have an active API that I can pull from or back-end developer resources to build one. As a result, I need to stub or mock up my data set so that the interfaces I create look real and you can see data changing but without the help of a back-end dev.
For this tutorial, I’m going to be making a zoo animal list view.
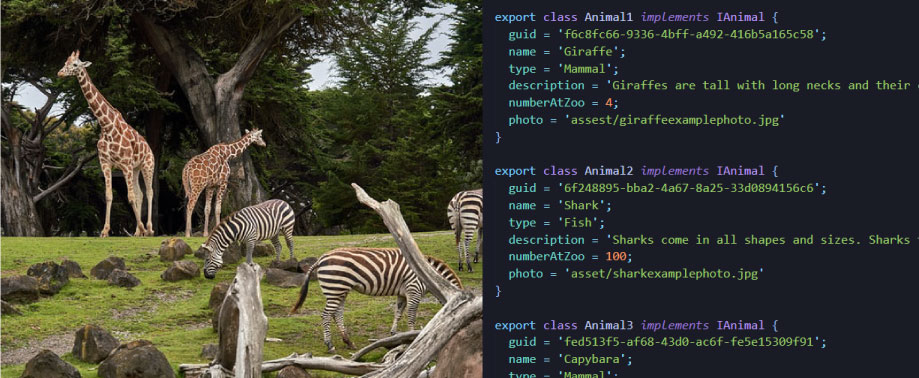
Animal List View
Starting with an angular project create a new component, use ng g c zoo-animals in the terminal.
ng g c zoo-animals
I’m are going to start by creating the UI for each component. Then once I have the mock data set I will move it out to be used in a more generic way across the app.
I go to the zoo-animails.component.ts file and export an interface of IAnimal so I can start building your data.
export interface IAnimal {
// Data will go here
}
Because I’m thinking about my animal with the context of being on the list view that’s the data I’m going to be most concerned with for this part of the series.
export interface IAnimal {
guid: string;
name: string;
type: string;
description: string;
numberAtZoo: number;
photo: string;
}
Ah, there that looks good, pretty straight forward. I’ve got a solid list of attributes that will appear in my list and identify the animals to the users.
I’ve defined in my interface that my classes are going to conform to this structure.
Next I export classes for each animal you want in my mock data. Then I fill in the class with the data conforming to the interface.
export class Animal1 implements IAnimal {
guid = 'f6c8fc66-9336-4bff-a492-416b5a165c58';
name = 'Giraffe';
type = 'Mammal';
description = 'Giraffes are tall with long necks and their coat has dark blotches or patches separated by light hair.';
numberAtZoo = 4;
photo = 'assest/giraffeexamplephoto.jpg'
}
export class Animal2 implements IAnimal {
guid = '6f248895-bba2-4a67-8a25-33d0894156c6';
name = 'Shark';
type = 'Fish';
description = 'Sharks come in all shapes and sizes. Sharks typically have sharp teeth, fins, and gills.',
numberAtZoo = 100;
photo = 'asset/sharkexamplephoto.jpg'
}
export class Animal3 implements IAnimal {
guid = 'fed513f5-af68-43d0-ac6f-fe5e15309f91';
name = 'Capybara';
type = 'Mammal';
description = 'Capybaras has a barrel-shaped body with a short head and reddish-brown fur.';
numberAtZoo = 4;
photo = 'asset/capybaraexamplephoto.jpg'
}
Animal Data for the List
Once I’ve got my data for 3 different animals ready to go I want to pull those into a list in the zoo-animals UI.
animals: IAnimal[] = [
new Animal1(),
new Animal2(),
new Animal3(),
];
For this view I prepared a property of animals that conforms to the animal interface from earlier. Notice I typed the IAnimal interface with the array syntax [ ]. This sets up our property to know that its going to take a list of instances of our animal classes.
Then I new up the three instances of our animal classes that I created above inside the array. Makes sense right, I want to create a list of animals so let’s give the interface an array!
Open your zoo-animals.component.html. For this project I’m using an Angular Material theme just for fun.
<code><table mat-table [dataSource]="animals" class="mat-elevation-z8">
<ng-container matColumnDef="name">
<th mat-header-cell *matHeaderCellDef> Name </th>
<td mat-cell *matCellDef="let animal"> {{animal.name}} </td>
</ng-container>
...
<tr mat-header-row *matHeaderRowDef="displayedColumns"></tr>
<tr mat-row *matRowDef="let row; columns: displayedColumns;"></tr>
</table></code>
As a result you can see I created my first column in the above code. Then I added the animal name and the “…” represent the remaining columns.
After that I needed to add the angular material way of defining the header and footer. Otherwise, you’ll get a nasty error. In the last two <tr> tags I reference an array of table names that match the matColumnDefinition on my ZooAnimalsComponent.
To finish it out I displayed the rest of the data defined in the interface for a user to know what they are clicking on to get to the detail page.
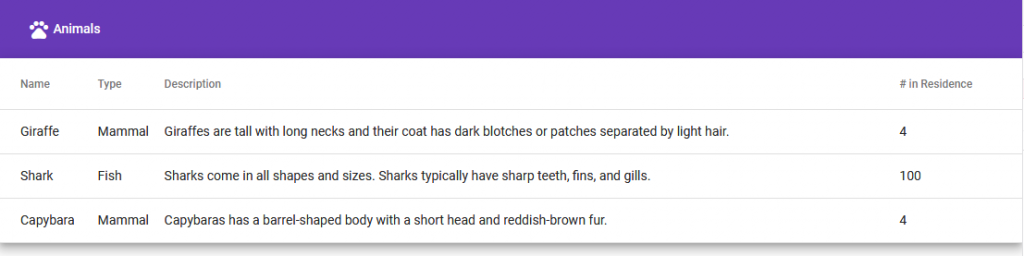
Link to the Correct Animal Detail View
Before I move on from this component I need to set up our link into the detail view.
<code> <ng-container matColumnDef="name">
<th mat-header-cell *matHeaderCellDef> Name </th>
<td mat-cell *matCellDef="let animal"><a [routerLink]="['/animals', animal.guid]"> {{animal.name}}</a> </td>
</ng-container> </code>
For this I’m adding a router link around my name column. I’m then planning on passing the following into the link. animals followed by the animal.guid property from each animal. Since I don’t have the animal component yet this doesn’t do anything but set us up for the next post in this series. In the next post, I will be diving into the detail view and all the mock data we will see there!
To wrap up
The work I did above will set me up nicely for diving into the detail page. It gives me a good starting point for my mock data and getting it displayed to the screen.
Coming soon!
- Part Two: Make mock data for your detail view component (Mock Data Series)
- Part Three: Mocking Data to be used in multiple components (Mock Data Series)
- Part Four: New Instances of your Animal class using the single point of data (Mock Data Series)
0 Comments